Comparing Deepseek V3 vs Qwen 2.5B - Case Study
We have experimented with Qwen 2.5B and Deepseek V3 as we challenge them with complex, multi-step problems. Join us for a deep dive into what these models can really do when the pressure is on.
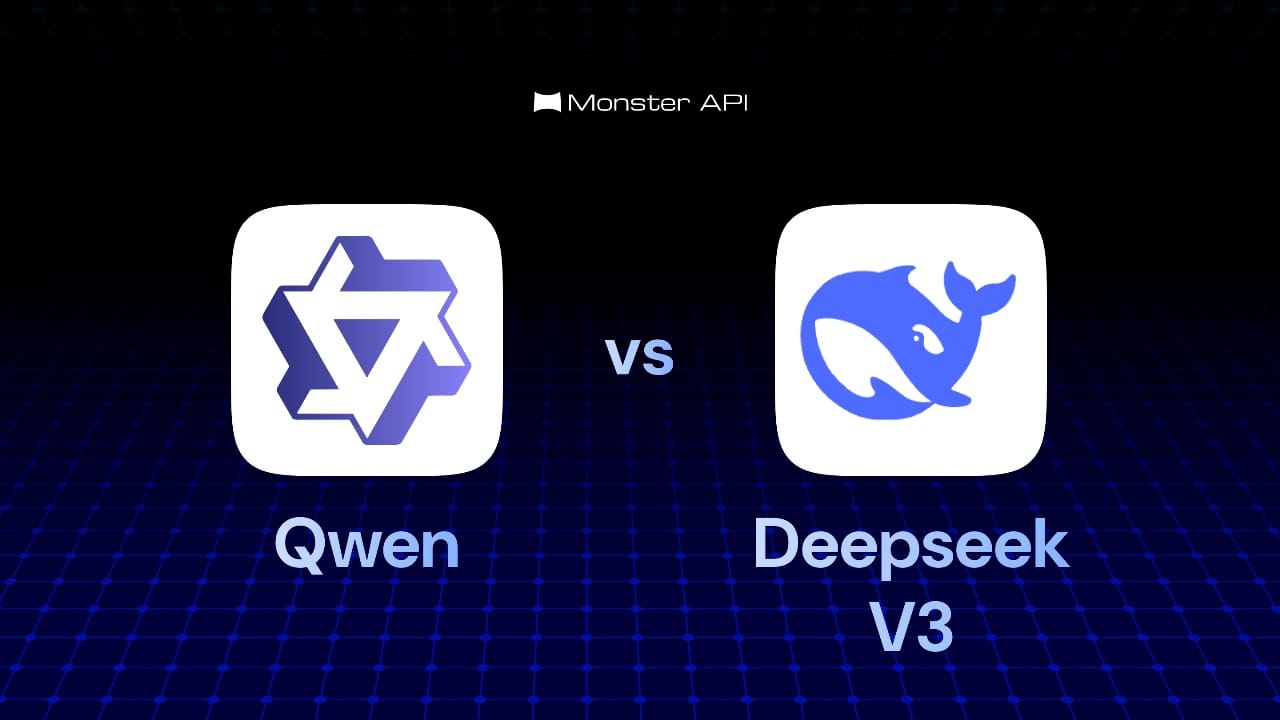
You know what’s wild about the AI space right now? Just when you think you’ve figured out which models are the best for specific tasks, something new drops and flips the whole game. That’s exactly how we felt when we started experimenting with Qwen 2 and Deepseek V3—especially when it comes to solving those tricky, multi-step problems.
In this blog, we are breaking down what we learned after putting these models through their paces with a bunch of different prompts. Whether you’re a developer trying to decide which model fits your project or just curious about how these AI “brains” work through challenges, we think you’ll find this pretty interesting. Let’s get into it and see how these two stack up when things get tough!
Methodology
To ensure a fair and comprehensive comparison, we developed specific test prompts for each parameter and evaluated both models using identical inputs. Each test was designed to assess not just basic capabilities but also edge cases and complex scenarios.
Parameters Tested
1. Code Generation
We tested both models' ability to generate code across different programming paradigms and complexity levels. Our test prompts included:
DeepSeek
- Creating a binary search tree implementation in Python
- Developing a responsive React component with TypeScript
- Writing complex SQL queries for business analytics
Key evaluation criteria:
- Syntax accuracy
- Implementation of best practices
- Error handling
- Code readability
- Documentation quality
Qwen
Prompt 1: Binary Search Tree in Python
To implement a binary search tree (BST), I need to define a Node class and then build methods for insertion, deletion, and finding the minimum value. Error handling will be crucial, especially for deletion and minimum value searches where the tree might be empty or nodes might not exist.
First, I'll define the Node class:
class Node:
def __init__(self, key):
self.left = None
self.right = None
self.val = key
Next, I'll create the BST class with insertion, deletion, and minimum value methods.
Insertion:
Insertion in a BST involves comparing the new value with the current node and deciding to go left or right until an empty spot is found.
Deletion:
Deletion is more complex and has three cases:
- Deleting a leaf node.
- Deleting a node with one child.
- Deleting a node with two children (need to find the in-order successor or predecessor).
Finding the Minimum Value:
The minimum value in a BST is the leftmost node.
I need to handle errors such as trying to delete a node that doesn't exist or finding the minimum in an empty tree.
I'll proceed to implement these methods with comments and error handling.
Final Solution
To solve this problem, we need to implement a binary search tree (BST) with the ability to insert, delete, and find the minimum value. We will also include proper error handling and comments for clarity.
Approach
- Node Class:
- Define a Node class to represent each node in the BST. Each node has a value and pointers to its left and right children.
- BST Class:
- Implement the following methods:
- insert(val): Inserts a new value into the BST.
- delete(val): Deletes a value from the BST.
- min_value(): Finds the minimum value in the BST.
- Use recursive approaches for insertion and deletion to handle the tree structure effectively.
- Include error handling to manage cases such as deleting a non-existent node or finding the minimum in an empty tree.
- Implement the following methods:
Solution Code
def __init__(self, key):
self.left = None
self.right = None
self.val = key
class BST:
def __init__(self):
self.root = None
def insert(self, val):
if not self.root:
self.root = Node(val)
else:
self._insert_recursive(self.root, val)
def _insert_recursive(self, current, val):
if val < current.val:
if current.left is None:
current.left = Node(val)
else:
self._insert_recursive(current.left, val)
elif val > current.val:
if current.right is None:
current.right = Node(val)
else:
self._insert_recursive(current.right, val)
else:
print("Value already exists in the tree.")
def delete(self, val):
if self.root is None:
print("Tree is empty.")
return
self.root = self._delete_recursive(self.root, val)
def _delete_recursive(self, current, val):
if current is None:
print("Value not found in the tree.")
return current
if val < current.val:
current.left = self._delete_recursive(current.left, val)
elif val > current.val:
current.right = self._delete_recursive(current.right, val)
else:
# Node with only one child or no child
if current.left is None:
return current.right
elif current.right is None:
return current.left
# Node with two children: Get the inorder successor (smallest in the right subtree)
temp_val = self._min_value_node(current.right).val
current.val = temp_val
current.right = self._delete_recursive(current.right, temp_val)
return current
def _min_value_node(self, node):
current = node
while current.left is not None:
current = current.left
return current
def min_value(self):
if self.root is None:
print("Tree is empty.")
return None
return self._min_value_node(self.root).val
# Example usage:
bst = BST()
bst.insert(50)
bst.insert(30)
bst.insert(20)
bst.insert(40)
bst.insert(70)
bst.insert(60)
bst.insert(80)
print("Minimum value:", bst.min_value())
bst.delete(20)
bst.delete(30)
bst.delete(50)
Explanation
- Insertion: We recursively find the correct position for the new value based on BST properties.
- Deletion: We handle three cases:
- The node is a leaf.
- The node has one child.
- The node has two children (replace with the inorder successor).
- Finding Minimum Value: We traverse to the leftmost node of the tree.
Prompt 2: React Component for Responsive Grid Layout
I need to create a React component that displays a grid of cards, each containing an image, title, and description. The grid should be responsive and include hover effects. Additionally, I need to use TypeScript for typing.
To approach this, I'll need to:
- Set up a basic React component with TypeScript.
- Create a card component that accepts props for image, title, and description.
- Style the grid to be responsive using CSS Grid or Flexbox.
- Add hover effects to the cards.
- Ensure proper typing for props and components.
I'll start by creating the card component and then the grid component that will render multiple cards.
Final Solution
To solve this problem, we need to create a responsive grid layout of cards in a React application using TypeScript. Each card should display an image, title, and description, and include hover effects.
Approach
- Create a Card Component:
- Define a Card component that accepts props for image source, title, and description.
- Style the card to display these elements appropriately.
- Create a Grid Component:
- Define a Grid component that renders a list of Card components.
- Use CSS Grid or Flexbox to create a responsive layout.
- Add hover effects to the cards for interactivity.
- Type Props with TypeScript:
- Ensure that all props are properly typed to maintain type safety.
Solution Code
First, create the Card component:
import React from 'react';
import './Card.css';
interface CardProps {
image: string;
title: string;
description: string;
}
const Card: React.FC<CardProps> = ({ image, title, description }) => {
return (
<div className="card">
<img src={image} alt={title} className="card-image" />
<div className="card-content">
<h3 className="card-title">{title}</h3>
<p className="card-description">{description}</p>
</div>
</div>
);
};
export default Card;
Next, create the Grid component:
// Grid.tsx
import React from 'react';
import Card from './Card';
import './Grid.css';
interface GridProps {
items: {
image: string;
title: string;
description: string;
}[];
}
const Grid: React.FC<GridProps> = ({ items }) => {
return (
<div className="grid">
{items.map((item, index) => (
<Card key={index} {...item} />
))}
</div>
);
};
export default Grid;
Add styles for the card:
/* Card.css */
.card {
border: 1px solid #ddd;
border-radius: 8px;
overflow: hidden;
box-shadow: 0 2px 5px rgba(0,0,0,0.1);
transition: transform 0.2s, box-shadow 0.2s;
}
.card:hover {
transform: translateY(-5px);
box-shadow: 0 4px 10px rgba(0,0,0,0.2);
}
.card-image {
width: 100%;
height: 200px;
object-fit: cover;
}
.card-content {
padding: 16px;
}
.card-title {
margin-top: 0;
color: #333;
}
.card-description {
color: #666;
}
Add styles for the grid:
/* Grid.css */
.grid {
display: grid;
grid-template-columns: repeat(auto-fill, minmax(250px, 1fr));
gap: 20px;
padding: 20px;
}
@media (max-width: 600px) {
.grid {
grid-template-columns: 1fr;
}
}
Explanation
- Card Component:
- Accepts image, title, and description as props.
- Styled with border, shadow, and hover effects for interactivity.
- Grid Component:
- Accepts an array of items, each with image, title, and description.
- Uses CSS Grid for responsive layout, adjusting based on screen size.
- Styling:
- Cards have transitions for smooth hover effects.
- Grid layout adapts to different screen sizes for responsiveness.
Prompt 3: SQL Query for Top Customers per Quarter
I need to write a SQL query to find the top 3 customers who have spent the most money in each quarter of 2023, including their contact details and total purchase amount. The database has tables for customers, orders, and order_items.
To approach this, I need to:
- Identify the structure of the tables involved:
- Customers table: likely has customer details like ID, name, contact info.
- Orders table: likely has order details including customer ID, order date.
- Order_items table: likely has individual items per order with quantities and prices.
- Calculate the total expenditure per customer per quarter.
- Rank customers within each quarter based on their total expenditure.
- Select the top 3 customers for each quarter.
- Include their contact details along with the total purchase amount.
I'll assume the following table structures for the sake of this exercise:
- Customers table:
- customer_id (primary key)
- name
- phone
- Orders table:
- order_id (primary key)
- customer_id (foreign key to Customers)
- order_date
- Order_items table:
- order_item_id (primary key)
- order_id (foreign key to Orders)
- product_id
- quantity
- price_per_unit
Given these structures, I'll need to join these tables to calculate the total expenditure per customer per quarter.
I can use window functions to rank customers within each quarter and then filter for the top 3 in each quarter.
I'll also need to ensure that the order dates are within 2023.
I should also consider edge cases, such as customers with the same expenditure in a quarter. In such cases, I might need to decide how to handle ties for the top positions.
For simplicity, I'll assume that if multiple customers have the same expenditure, all are included in the top list for that quarter.
Final Solution
To solve this problem, we need to identify the top 3 customers who have spent the most money in each quarter of 2023, including their contact details and total purchase amount. We will achieve this by writing a SQL query that joins the Customers, Orders, and Order_items tables, calculates the total expenditure per customer per quarter, ranks them accordingly, and selects the top 3 for each quarter.
Approach
- Join Tables:
- Join the Customers, Orders, and Order_items tables to access customer details and their respective orders and order items.
- Calculate Expenditure:
- Calculate the total expenditure per customer per quarter by summing the product of quantity and price_per_unit for each order item.
- Rank Customers:
- Use window functions to rank customers within each quarter based on their total expenditure.
- Select Top Customers:
- Filter to include only the top 3 customers for each quarter.
- Include Customer Details:
- Retrieve and display customer contact details along with their total purchase amount.
Solution Code
quarter,
c.customer_id,
c.name,
c.email,
c.phone,
total_amount
FROM
(SELECT
customer_id,
DATE_TRUNC('quarter', o.order_date) AS quarter,
SUM(oi.quantity * oi.price_per_unit) AS total_amount,
RANK() OVER (PARTITION BY DATE_TRUNC('quarter', o.order_date) ORDER BY SUM(oi.quantity * oi.price_per_unit) DESC) AS customer_rank
FROM
Orders o
JOIN
Order_items oi ON o.order_id = oi.order_id
WHERE
EXTRACT(YEAR FROM o.order_date) = 2023
GROUP BY
customer_id, DATE_TRUNC('quarter', o.order_date)
) AS quarterly_sales
JOIN
Customers c ON quarterly_sales.customer_id = c.customer_id
WHERE
customer_rank <= 3
ORDER BY
quarter,
total_amount DESC;
Explanation
- Joining Tables:
- We join the Orders and Order_items tables to calculate the total expenditure per customer per quarter.
- Calculating Expenditure:
- We use DATE_TRUNC('quarter', o.order_date) to group orders by quarter.
- We sum the product of quantity and price_per_unit for each order item to get the total expenditure per customer per quarter.
- Ranking Customers:
- We use the RANK() window function to rank customers within each quarter based on their total expenditure.
- Selecting Top Customers:
- We filter the results to include only those customers who have a rank of 3 or less in their respective quarters.
- Including Customer Details:
- We join the Customers table to retrieve and display customer details such as name, email, and phone.
This query ensures that we identify and retrieve the top spenders for each quarter in 2023, providing a clear view of customer expenditure and contact information for further action.
2. Logical Reasoning
To assess logical reasoning capabilities, we presented both models with structured puzzles and probability problems. Test cases included:
DeepSeek
- Prompt 1: "Three friends - Alice, Bob, and Charlie - are sitting in a row at a movie theater. We know that: Alice is not sitting at either end. Bob is not sitting next to Charlie. Who is sitting where? Explain your reasoning."
- Prompt 2: "In a drawer, there are red socks, blue socks, and black socks in a ratio of 4:2:3. If I reach in without looking and take out two socks, what is the probability that they match? Show your work."
Qwen
Prompt 1: Seating Arrangement
We have three friends: Alice, Bob, and Charlie, sitting in a row at a movie theater. The conditions are:
- Alice is not sitting at either end.
- Bob is not sitting next to Charlie.
We need to determine who is sitting where.
Let's analyze this step by step.
First, since there are only three seats in a row, let's label them as Seat 1, Seat 2, and Seat 3.
Given that Alice is not sitting at either end, she cannot be in Seat 1 or Seat 3. Therefore, Alice must be in Seat 2.
So, the seating arrangement looks like this:
Seat 1: ?
Seat 2: Alice
Seat 3: ?
Now, the second condition is that Bob is not sitting next to Charlie.
Since Alice is in Seat 2, the remaining seats are Seat 1 and Seat 3, which are next to each other.
If we place Bob in Seat 1 and Charlie in Seat 3, they would be sitting next to each other, which violates the condition.
Similarly, if we place Charlie in Seat 1 and Bob in Seat 3, they would still be sitting next to each other, again violating the condition.
Wait a minute, in a row of three seats, Seats 1 and 3 are next to Seat 2, which is occupied by Alice. But Seats 1 and 3 are also next to each other.
Given that, is there a way to place Bob and Charlie such that they are not sitting next to each other?
Let's think about it.
If Alice is in Seat 2, and we place Bob in Seat 1, then Charlie must not be in Seat 3, because then Bob and Charlie would be next to each other.
Similarly, if we place Charlie in Seat 1, then Bob cannot be in Seat 3.
So, to satisfy the condition that Bob is not sitting next to Charlie, neither Bob nor Charlie can be in Seats 1 and 3 simultaneously.
Wait, but Seats 1 and 3 are the only two seats available, and they are next to each other and next to Alice.
This seems tricky.
Let me consider that maybe the condition cannot be satisfied, or perhaps I'm missing something.
Alternatively, maybe the condition implies that Bob and Charlie cannot both be sitting next to Alice.
But that doesn't seem right.
Wait, perhaps I need to consider that in a row of three seats, it's impossible to have Bob and Charlie not sitting next to each other because the only arrangement is that one is at the end and the other in the middle, but since Alice is in the middle, the ends are next to each other.
This is confusing.
Let me try another approach.
If Alice is in Seat 2, and Bob is not sitting next to Charlie, perhaps one of them has to be at one end, and the other has to be somewhere else, but in a row of three, that's not possible without them being next to each other.
Wait, perhaps the condition is impossible to satisfy given the first condition.
Alternatively, maybe the initial assumption that Alice is in Seat 2 is correct, and then we have to see if the second condition can be met.
But in a row of three seats, if Alice is in the middle, and Bob and Charlie are on the ends, they have to be next to Alice, but not necessarily next to each other unless the seats are arranged in a way that the ends are adjacent.
Wait, in a straight row, seats 1 and 3 are adjacent to seat 2, but are seats 1 and 3 adjacent to each other?
Yes, in a row of three seats, seats 1 and 3 are adjacent to seat 2, and seats 1 and 3 are also adjacent to each other.
Wait, no, in a row, seat 1 is only adjacent to seat 2, and seat 3 is only adjacent to seat 2. Seats 1 and 3 are not directly adjacent to each other.
Wait, but in a straight row of three seats, seats 1 and 3 are both adjacent to seat 2, but they are not adjacent to each other.
Is that correct?
Let me visualize it:
Seat 1 - Seat 2 - Seat 3
So, seat 1 is next to seat 2, seat 2 is next to seat 3.
Therefore, seats 1 and 3 are not directly next to each other; they are both next to seat 2.
Given that, if Bob is in seat 1 and Charlie is in seat 3, they are not sitting next to each other; they are both sitting next to Alice.
Similarly, if Charlie is in seat 1 and Bob is in seat 3, same situation.
So, in this arrangement, Bob and Charlie are not sitting next to each other; they are sitting on either side of Alice.
Therefore, the condition is satisfied.
So, the arrangement is:
Seat 1: Bob or Charlie
Seat 2: Alice
Seat 3: Charlie or Bob
Thus, there are two possible arrangements:
- Seat 1: Bob, Seat 2: Alice, Seat 3: Charlie
- Seat 1: Charlie, Seat 2: Alice, Seat 3: Bob
In both cases, Bob and Charlie are not sitting next to each other; they are sitting on either side of Alice.
Therefore, either arrangement satisfies both conditions.
Final Solution
Given the conditions:
- Alice is not sitting at either end.
- Bob is not sitting next to Charlie.
The seating arrangement is as follows:
- Alice must sit in the middle seat.
- Bob and Charlie can sit in the end seats, but they cannot sit next to each other.
Given that the row has three seats, with Alice in the middle, Bob and Charlie can each sit in one of the end seats without being next to each other.
Thus, the possible seating arrangements are:
- Seat 1: Bob, Seat 2: Alice, Seat 3: Charlie
- Seat 1: Charlie, Seat 2: Alice, Seat 3: Bob
Both arrangements satisfy the given conditions.
Probability of Drawing Two Matching Socks
In a drawer, there are red socks, blue socks, and black socks in a ratio of 4:2:3. If two socks are drawn at random, what is the probability that they match?
Let's denote the number of red socks as 4k, blue socks as 2k, and black socks as 3k, where k is a positive integer.
Total number of socks = 4k + 2k + 3k = 9k.
The total number of ways to draw two socks from 9k socks is:
[ \binom{9k}{2} = \frac{9k(9k - 1)}{2} ]
Next, we calculate the number of ways to draw two socks of the same color.
- Red socks:
Number of ways to choose two red socks:
[ \binom{4k}{2} = \frac{4k(4k - 1)}{2} ]
- Blue socks:
Number of ways to choose two blue socks:
[ \binom{2k}{2} = \frac{2k(2k - 1)}{2} ]
- Black socks:
Number of ways to choose two black socks:
[ \binom{3k}{2} = \frac{3k(3k - 1)}{2} ]
The total number of ways to draw two matching socks is the sum of the above:
[ \frac{4k(4k - 1)}{2} + \frac{2k(2k - 1)}{2} + \frac{3k(3k - 1)}{2} ]
Simplify each term:
[ \frac{4k(4k - 1)}{2} = 8k^2 - 2k ]
[ \frac{2k(2k - 1)}{2} = 2k^2 - k ]
[ \frac{3k(3k - 1)}{2} = \frac{9k^2 - 3k}{2} = 4.5k^2 - 1.5k ]
Sum these up:
[ 8k^2 - 2k + 2k^2 - k + 4.5k^2 - 1.5k = (8k^2 + 2k^2 + 4.5k^2) + (-2k - k - 1.5k) = 14.5k^2 - 4.5k ]
Now, the probability P that the two socks match is the number of matching pairs divided by the total number of pairs:
[ P = \frac{14.5k^2 - 4.5k}{\frac{9k(9k - 1)}{2}} = \frac{14.5k^2 - 4.5k}{\frac{81k^2 - 9k}{2}} = \frac{14.5k^2 - 4.5k}{40.5k^2 - 4.5k} ]
Simplify the numerator and denominator:
Numerator: (14.5k^2 - 4.5k = k(14.5k - 4.5))
Denominator: (40.5k^2 - 4.5k = k(40.5k - 4.5))
Thus,
[ P = \frac{k(14.5k - 4.5)}{k(40.5k - 4.5)} = \frac{14.5k - 4.5}{40.5k - 4.5} ]
Factor out 0.5 from numerator and denominator:
[ P = \frac{0.5(29k - 9)}{0.5(81k - 9)} = \frac{29k - 9}{81k - 9} ]
Factor out 9 from the denominator:
[ P = \frac{29k - 9}{9(9k - 1)} ]
This is the probability in terms of k. However, since k is a common factor and the ratio is fixed, we can consider k=1 for simplicity, but the expression is already simplified.
Alternatively, to get a numerical probability, we can consider the ratio of the coefficients for large k, where the lower order terms become negligible:
For large k:
[ P \approx \frac{29k}{81k} = \frac{29}{81} ]
Thus, the probability that the two socks match is approximately (\frac{29}{81}).
However, for exact calculation, we can leave it as (\frac{29k - 9}{81k - 9}), but since k is a positive integer, for k=1:
[ P = \frac{29(1) - 9}{81(1) - 9} = \frac{20}{72} = \frac{5}{18} ]
For k=2:
[ P = \frac{29(2) - 9}{81(2) - 9} = \frac{58 - 9}{162 - 9} = \frac{49}{153} ]
Wait, this suggests that the probability changes with k, but in reality, since the ratio of socks is fixed, the probability should be consistent regardless of k.
Let me recast this.
Given the ratio of red : blue : black = 4:2:3, the total parts are 4 + 2 + 3 = 9 parts.
Let’s consider the proportion of each color:
- Red: (\frac{4}{9})
- Blue: (\frac{2}{9})
- Black: (\frac{3}{9} = \frac{1}{3})
The probability of drawing two socks of the same color is the sum of the probabilities of drawing two reds, two blues, or two blacks.
The formula for the probability of drawing two matching socks is:
[ P = \frac{\text{number of ways to choose two socks of the same color}}{\text{total number of ways to choose any two socks}} ]
Using combinations:
[ P = \frac{\binom{4}{2} + \binom{2}{2} + \binom{3}{2}}{\binom{9}{2}} ]
Calculate each combination:
[ \binom{4}{2} = 6, \quad \binom{2}{2} = 1, \quad \binom{3}{2} = 3 ]
[ \binom{9}{2} = 36 ]
So,
[ P = \frac{6 + 1 + 3}{36} = \frac{10}{36} = \frac{5}{18} ]
Therefore, the probability that the two socks match is (\frac{5}{18}).
Final Solution
Seating Arrangement
Given the conditions:
- Alice is not sitting at either end.
- Bob is not sitting next to Charlie.
The seating arrangement is as follows:
- Alice must sit in the middle seat.
- Bob and Charlie can sit in the end seats.
Thus, the possible seating arrangements are:
- Seat 1: Bob, Seat 2: Alice, Seat 3: Charlie
- Seat 1: Charlie, Seat 2: Alice, Seat 3: Bob
Both arrangements satisfy the given conditions.
Probability of Drawing Two Matching Socks
Given the ratio of red : blue : black socks is 4:2:3, the total parts sum to 9.
The probability that two randomly drawn socks match is calculated as:
[ P = \frac{\binom{4}{2} + \binom{2}{2} + \binom{3}{2}}{\binom{9}{2}} = \frac{6 + 1 + 3}{36} = \frac{10}{36} = \frac{5}{18} ]
Therefore, the probability is ( \boxed{\dfrac{5}{18}} ).
We evaluated:
- Step-by-step reasoning
- Clarity of explanations
- Accuracy of conclusions
- Handling of edge cases
3. Mathematical Reasoning
The mathematical reasoning assessment focused on both practical and theoretical mathematics:
DeepSeek
Prompt 1: "A company's revenue grew by 15% in 2021, 23% in 2022, and declined by 8% in 2023. If their revenue in 2020 was $1 million, what was their revenue in 2023? Show all calculations."
Prompt 2: "Solve this calculus problem and explain each step: Find the volume of the solid formed by rotating the region bounded by y = x², y = 2x, and the y-axis around the x-axis."
Qwen
Prompt 1: Company Revenue Calculation
The company's revenue in 2020 was $1 million. In 2021, it grew by 15%, in 2022 by 23%, and in 2023 it declined by 8%. I need to find the revenue in 2023.
First, let's calculate the revenue for each year step by step.
2021:
Starting revenue: $1,000,000
Growth: 15%
Revenue in 2021 = Starting revenue + (Growth percentage * Starting revenue)
= $1,000,000 + (0.15 * $1,000,000)
= $1,000,000 + $150,000
= $1,150,000
Alternatively, we can calculate it as:
Revenue in 2021 = Starting revenue * (1 + Growth percentage)
= $1,000,000 * (1 + 0.15)
= $1,000,000 * 1.15
= $1,150,000
2022:
Starting revenue: $1,150,000
Growth: 23%
Revenue in 2022 = Revenue in 2021 * (1 + Growth percentage)
= $1,150,000 * (1 + 0.23)
= $1,150,000 * 1.23
= $1,414,500
2023:
Starting revenue: $1,414,500
Decline: 8%
Since it's a decline, the percentage is subtracted.
Revenue in 2023 = Revenue in 2022 * (1 - Decline percentage)
= $1,414,500 * (1 - 0.08)
= $1,414,500 * 0.92
= $1,302,420
So, the revenue in 2023 was $1,302,420.
Prompt 2: Calculus Problem - Volume of Solid of Revolution
The problem is to find the volume of the solid formed by rotating the region bounded by y = x², y = 2x, and the y-axis around the x-axis.
To solve this, I'll use the method of disks or washers, since we're rotating around the x-axis.
First, I need to find the points of intersection of the curves y = x² and y = 2x to determine the limits of integration.
Set x² = 2x
x² - 2x = 0
x(x - 2) = 0
So, x = 0 and x = 2
Therefore, the limits of integration are from x = 0 to x = 2.
Next, I need to determine which function is above the other in this interval.
For x in [0, 2], 2x > x², so y = 2x is the upper function.
The volume V of the solid of revolution is given by the washer method:
V = π ∫a to b^2 - (r(x))^2 dx
Where R(x) is the outer radius and r(x) is the inner radius.
In this case, since we're rotating around the x-axis:
- The outer radius R(x) is the distance from the x-axis to y = 2x, which is 2x.
- The inner radius r(x) is the distance from the x-axis to y = x², which is x².
Therefore, the volume is:
V = π ∫0 to 2^2 - (x²)^2 dx
= π ∫[0 to 2](4x² - x⁴) dx
Now, integrate term by term:
= π [ (4/3)x³ - (1/5)x⁵ ] evaluated from 0 to 2
Plug in the limits:
= π [ (4/3)(2)^3 - (1/5)(2)^5 ] - π [ (4/3)(0)^3 - (1/5)(0)^5 ]
= π [ (4/3)(8) - (1/5)(32) ] - π [0]
= π [ 32/3 - 32/5 ]
To subtract these fractions, find a common denominator, which is 15:
= π [ (32/3)(5/5) - (32/5)(3/3) ]
= π [ 160/15 - 96/15 ]
= π [ 64/15 ]
Therefore, the volume is:
V = 64π/15
So, the volume of the solid is 64π/15 cubic units.
Final Solution
Revenue Calculation
Given the revenue in 2020 was $1,000,000, with growth rates of 15% in 2021, 23% in 2022, and a decline of 8% in 2023, the revenue in 2023 is calculated as follows:
- 2021 Revenue: $1,000,000 * 1.15 = $1,150,000
- 2022 Revenue: $1,150,000 * 1.23 = $1,414,500
- 2023 Revenue: $1,414,500 * 0.92 = $1,302,420
Thus, the revenue in 2023 is $\boxed{1,302,420}$.
Calculus Problem - Volume of Solid of Revolution
To find the volume of the solid formed by rotating the region bounded by y = x², y = 2x, and the y-axis around the x-axis, we use the washer method.
- Find the points of intersection:Set y = x² equal to y = 2x:[ x^2 = 2x \implies x^2 - 2x = 0 \implies x(x - 2) = 0 \implies x = 0, 2 ]
- Determine the outer and inner radii:
- Outer radius ( R(x) = 2x )
- Inner radius ( r(x) = x^2 )
- Set up the integral for volume:[ V = \pi \int_{0}^{2} (R(x))^2 - (r(x))^2 , dx = \pi \int_{0}^{2} (4x^2 - x^4) , dx ]
- Evaluate the integral:[ V = \pi \left[ \frac{4}{3}x^3 - \frac{1}{5}x^5 \right]_{0}^{2} = \pi \left( \frac{4}{3}(8) - \frac{1}{5}(32) \right) - \pi (0) = \pi \left( \frac{32}{3} - \frac{32}{5} \right) ]
- Simplify the expression:[ \frac{32}{3} - \frac{32}{5} = \frac{160}{15} - \frac{96}{15} = \frac{64}{15} ]Therefore, ( V = \pi \times \frac{64}{15} = \frac{64\pi}{15} )
Thus, the volume of the solid is ( \boxed{\dfrac{64\pi}{15}} ).
Evaluation criteria included:
- Calculation accuracy
- Show of work
- Mathematical notation usage
- Explanation clarity
4. Multilingual Capabilities
We tested language proficiency across multiple languages and content types:
Prompt 1: "Translate this business email to Spanish, French, and Mandarin Chinese while maintaining a professional tone:
'Dear valued client,
Thank you for your recent inquiry about our services. We would be happy to schedule a meeting next week to discuss your requirements in detail. Please let me know your preferred time and date.
Best regards,
John Smith'"
Prompt 2: "Write a short story about a lost cat finding its way home. The story should be about 100 words. Provide versions in English, Japanese, and German. Ensure the tone and meaning remain consistent across all languages."
DeepSeeK
Qwen
Prompt 1: Business Email Translation
The original email is:
"Dear valued client,
Thank you for your recent inquiry about our services. We would be happy to schedule a meeting next week to discuss your requirements in detail. Please let me know your preferred time and date.
Best regards,
John Smith"
Spanish Translation
"Estimado cliente valorado,
Gracias por su reciente consulta sobre nuestros servicios. Sería un placer programar una reunión la próxima semana para discutir sus requisitos en detalle. Por favor, háganos saber su tiempo y fecha preferidos.
Atentamente,
Juan Smith"
French Translation
"Cher client,
Merci pour votre récente demande concernant nos services. Nous serions ravis de programmer une réunion la semaine prochaine pour discuter de vos besoins en détail. Veuillez nous informer de votre date et heure préférées.
Meilleures salutations,
Jean Smith"
Mandarin Chinese Translation
"尊敬的客户,
感谢您最近对我们服务的查询。我们很乐意在下周安排一次会议,详细讨论您的需求。请告知您首选的时间和日期。
此致
敬礼
约翰·史密斯"
Prompt 2: Short Story in Multiple Languages
English Version
In the quiet town of Meadowgrove, Whiskers, a gray tabby cat, wandered off during a family picnic. Panicked searches turned up nothing, and as dusk fell, hopes dimmed. But Whiskers, resourceful and determined, followed familiar scents and sounds. Past the old oak tree, through the whispers of leaves, he navigated the night. Starlight guided his path, and by dawn, he was back, weary but home at last.
Japanese Version
メドウグローブの静かな町で、家族のピクニック中にグレイタビーの猫のウィスカーズが迷子になりました。慌てて探したけれど見つからず、夜が迫るにつれ希望は薄れていました。しかし、ウィスカーズは賢く頑強で、 familiar scents and soundsに沿って進みました。古いオークの木のそばを通って、葉のささやきに導かれ、夜路を進みました。星の光が彼の道を照らし、明け方にはついに家に帰ってきました。疲れてはいましたが、やっと家に戻ったのです。
German Version
In der ruhigen Stadt Meadowgrove-wanderte Whiskers, eine graue Streifkatze, während eines Familienpicknicks ab. Panische Suchen ergaben nichts, und als der Abend hereinbrach, verblassten die Hoffnungen. Doch Whiskers, gerissen und entschlossen, folgte vertrauten Gerüchen und Geräuschen. An dem alten Eichenbaum vorbei, durch das Flüstern der Blätter, navigierte er die Nacht. Sternenlicht leitete seinen Weg, und bei Tagesanbruch war er zurück, müde, aber endlich zu Hause.
Languages tested:
- English
- Spanish
- French
- Mandarin Chinese
- Japanese
- German
Key aspects evaluated:
- Grammar accuracy
- Cultural nuances
- Tone consistency
- Technical vocabulary
5. Copywriting
The copywriting assessment focused on creating marketing content across different formats:
Prompt 1: "Write compelling product descriptions for a new smartwatch with these features:
48-hour battery life
Heart rate monitoring
Sleep tracking
Water resistance (50m)
Social media notifications
Write both a short (50 words) and long (200 words) version targeting tech-savvy professionals."
Prompt 2: "Create a social media campaign (3 posts) for a local coffee shop that's introducing seasonal autumn drinks. Include hashtags and emotional hooks. Each post should highlight different aspects: ambiance, drinks, and special offers."
DeepSeek
Qwen
Prompt 1 : Product descriptions for a new smartwatch
Writing product descriptions for a new smartwatch. The target audience is tech-savvy professionals, and there are specific features to highlight: 48-hour battery life, heart rate monitoring, sleep tracking, water resistance up to 50 meters, and social media notifications. I need to write both a short and a long version of the description.
Starting with the short version, which should be around 50 words. Since it's brief, I need to make every word count. I should probably mention the key features and perhaps emphasize the convenience it offers to busy professionals. Something like:
"Meet your ultimate companion: a smartwatch designed for efficiency and style. Enjoy uninterrupted use with a robust 48-hour battery life. Stay connected with social media notifications, monitor your health with heart rate and sleep tracking, and embrace an active lifestyle with water resistance up to 50 meters. Elevate your professionalism and wellness today."
That's about 50 words. It covers the main points and tries to appeal to the professionalism of the target audience.
Now, for the long version, around 200 words. Here, I can expand on each feature and provide more details about how the smartwatch benefits the user. I should also consider the lifestyle of tech-savvy professionals and how this device can enhance their daily routines.
"Introducing the ultimate smartwatch tailored for tech-savvy professionals who demand performance and style in equal measure. This cutting-edge accessory is designed to keep you connected, informed, and healthy, all in one sleek package.
At the heart of this smartwatch is a powerful yet energy-efficient system that delivers an impressive 48-hour battery life. Say goodbye to constant charging and hello to uninterrupted use, whether you're powering through meetings or tackling your to-do list. No more worrying about your device dying at the most inopportune moments.
Stay on top of your health with advanced heart rate monitoring and comprehensive sleep tracking. Keep tabs on your cardiovascular wellness throughout the day and get insights into your sleep patterns to ensure you're rested and ready to conquer each new day. The smartwatch's water resistance up to 50 meters means you can swim, shower, or splash around without worrying about damaging your device.
Connectivity is seamless with social media notifications right on your wrist. Never miss an important message or update again. Receive alerts directly from your favorite platforms, allowing you to stay connected without constantly reaching for your phone.
Crafted with precision and durability in mind, this smartwatch is built to withstand the demands of an active lifestyle. Its water resistance not only allows for swimming but also ensures it can handle whatever life throws your way, from sweat-inducing workouts to accidental spills.
Elegantly designed to complement any professional attire, this smartwatch is not just a gadget—it's a statement of your sophistication and commitment to efficiency. Whether you're in the boardroom or on the go, this smartwatch seamlessly integrates into your lifestyle, offering functionality and fashion in perfect harmony.
Invest in a smarter way to stay connected and healthy. Upgrade to this exceptional smartwatch today and experience the future of wearable technology tailored just for you."
That should be around 200 words. I tried to elaborate on each feature and relate it to the benefits for the user, emphasizing convenience, health monitoring, and style.
Prompt 2 : Create a social media campaign
Creating a social media campaign for a local coffee shop introducing seasonal autumn drinks. I need to create three posts that highlight different aspects: ambiance, drinks, and special offers. Each post should include hashtags and emotional hooks to engage the audience.
First, let's think about the ambiance. Autumn is a season that often invokes feelings of warmth and coziness. People associate it with crisp air, falling leaves, and comforting interiors. So, for the ambiance post, I should focus on creating an inviting atmosphere that people would want to escape to during the cooler months.
Post 1:
"🍃 Fall into Coziness 🍁
Escape to our cozy corner with plush seating and flickering lights. Ideal for sipping on your favorite autumn brew while enjoying the serene atmosphere. Perfect for a quiet read or a catch-up with friends.
htag #CozyCorner #FallVibes #LocalCoffeeLove"
This post aims to evoke feelings of comfort and relaxation, encouraging followers to visit the coffee shop for a pleasant experience beyond just the drinks.
Next, highlighting the drinks themselves. Seasonal beverages are a big draw, and people are often eager to try new flavors that match the season. For this post, I should describe the drinks in a way that makes them sound enticing and irresistible.
Post 2:
"☕️ Sip into Autumn ☕️
Indulge in our new seasonal drinks, crafted to warm your soul on chilly days. From Spiced Caramel Lattes to Pumpkin Spice Cold Brews, each sip is a flavor explosion perfect for this time of year.
#SipIntoAutumn #SeasonalFavorites #CoffeeLovers"
Here, I'm trying to make the drinks sound delicious and unique, using descriptive phrases like "flavor explosion" to pique interest.
Lastly, promoting special offers to entice customers to try the new drinks or visit more frequently. People often respond well to deals and rewards, so this post should offer something extra for their visit.
Post 3:
"🍁 Fall in Love with Savings 🍁
Visit us this fall and enjoy exclusive discounts on our new seasonal drinks. Plus, sign up for our loyalty program and earn points towards free drinks all season long!
#FallDiscounts #LoyaltyReward #CoffeeSavings"
This post not only offers immediate savings but also encourages long-term engagement through a loyalty program.
Overall, these three posts cover different angles to attract customers: the inviting atmosphere, delicious drinks, and attractive offers. Each post should resonate with the target audience and encourage them to visit the coffee shop and try the new autumn drinks.
Final Solution
Product Descriptions for Smartwatch
Short Version (50 words)
"Meet your ultimate companion: a smartwatch designed for efficiency and style. Enjoy uninterrupted use with a robust 48-hour battery life. Stay connected with social media notifications, monitor your health with heart rate and sleep tracking, and embrace an active lifestyle with water resistance up to 50 meters. Elevate your professionalism and wellness today."
Long Version (200 words)
"Introducing the ultimate smartwatch tailored for tech-savvy professionals who demand performance and style in equal measure. This cutting-edge accessory is designed to keep you connected, informed, and healthy, all in one sleek package.
At the heart of this smartwatch is a powerful yet energy-efficient system that delivers an impressive 48-hour battery life. Say goodbye to constant charging and hello to uninterrupted use, whether you're powering through meetings or tackling your to-do list. No more worrying about your device dying at the most inopportune moments.
Stay on top of your health with advanced heart rate monitoring and comprehensive sleep tracking. Keep tabs on your cardiovascular wellness throughout the day and get insights into your sleep patterns to ensure you're rested and ready to conquer each new day. The smartwatch's water resistance up to 50 meters means you can swim, shower, or splash around without worrying about damaging your device.
Connectivity is seamless with social media notifications right on your wrist. Never miss an important message or update again. Receive alerts directly from your favorite platforms, allowing you to stay connected without constantly reaching for your phone.
Crafted with precision and durability in mind, this smartwatch is built to withstand the demands of an active lifestyle. Its water resistance not only allows for swimming but also ensures it can handle whatever life throws your way, from sweat-inducing workouts to accidental spills.
Elegantly designed to complement any professional attire, this smartwatch is not just a gadget—it's a statement of your sophistication and commitment to efficiency. Whether you're in the boardroom or on the go, this smartwatch seamlessly integrates into your lifestyle, offering functionality and fashion in perfect harmony.
Invest in a smarter way to stay connected and healthy. Upgrade to this exceptional smartwatch today and experience the future of wearable technology tailored just for you."
Social Media Campaign for Local Coffee Shop
Post 1: Ambiance
"🍃 Fall into Coziness 🍁
Escape to our cozy corner with plush seating and flickering lights. Ideal for sipping on your favorite autumn brew while enjoying the serene atmosphere. Perfect for a quiet read or a catch-up with friends.
#CozyCorner #FallVibes #LocalCoffeeLove"
Post 2: Drinks
"☕️ Sip into Autumn ☕️
Indulge in our new seasonal drinks, crafted to warm your soul on chilly days. From Spiced Caramel Lattes to Pumpkin Spice Cold Brews, each sip is a flavor explosion perfect for this time of year.
#SipIntoAutumn #SeasonalFavorites #CoffeeLovers"
Post 3: Special Offers
"🍁 Fall in Love with Savings 🍁
Visit us this fall and enjoy exclusive discounts on our new seasonal drinks. Plus, sign up for our loyalty program and earn points towards free drinks all season long!
#FallDiscounts #LoyaltyReward #CoffeeSavings"
We evaluated:
- Persuasive writing
- Brand voice consistency
- Emotional engagement
- Call-to-action effectiveness
Testing Process
For each parameter, we:
- Used identical prompts for both models
- Recorded response times
- Evaluated output quality
- Documented any notable differences
- Tested multiple iterations where applicable
Results
Qwen and DeepSeek have distinctly different approaches to handling tasks. Think of Qwen as that thorough friend who loves explaining things in detail - they'll break everything down and consider all possible angles. They're big on documentation, walking you through their thought process, and making sure you understand every step.
DeepSeek, on the other hand, is more like your straight-to-the-point friend who gets things done efficiently. They'll give you what you need without the extra details.
In coding, Qwen builds things like they're planning for the future - lots of comments, error checks, and room for expansion. DeepSeek keeps it simple and functional - they'll handle the basics well but won't overcomplicate things.
For math and problem-solving, Qwen's like a detailed math tutor, explaining every step and assumption. DeepSeek's more like a study buddy who shows you the key steps to get to the answer.
When it comes to languages and writing, Qwen gets into the cultural nuances and tells engaging stories. DeepSeek keeps translations accurate but straightforward, and their writing focuses on the key facts.
For user interfaces, Qwen thinks about everything - accessibility, responsiveness, the works. DeepSeek makes sure it works well but keeps things basic.
In terms of optimization, Qwen plans ahead for performance from the start. DeepSeek's philosophy is more "let's get it working first, then we can make it faster."
The bottom line? Qwen's your go-to for detailed, well-documented solutions with all the bells and whistles. DeepSeek's perfect when you need something that works well and gets straight to the point.
Future Considerations
As both models continue to evolve, it's important to:
- Regularly rerun comparisons with updated versions
- Adapt test cases to new capabilities
- Consider emerging use cases and requirements
- Track performance improvements over time
Conclusion
This experimental framework provides a structured approach to comparing Qwen and DeepSeek across critical parameters. By using these prompts and evaluation criteria, developers and organizations can make informed decisions based on their specific needs and use cases.
Remember that model performance can vary based on specific use cases, and what works best for one application might not be optimal for another. Regular testing and evaluation are recommended as these models continue to evolve.